Does the seemingly indecipherable string of characters hold the key to unlocking a world of information? The ability to understand and manipulate text, regardless of its origin or encoding, is a cornerstone of modern computing and a vital skill in an increasingly interconnected world.
The challenge presented is to decipher the meaning and significance of a series of seemingly random characters. These characters, as it turns out, are not random at all. They represent Arabic text, albeit in a form that is not immediately readable to those unfamiliar with the language. The task is to convert this encoded text into a form that is both understandable and usable, specifically through the application of C# code.
The first step involves recognizing that the input is in a particular encoding. Based on the provided information, we know that the characters are Arabic text, but their representation suggests that it is not standard UTF-8 encoding that is commonly used for websites. This indicates that these characters are represented in a format that is not directly human-readable. The primary task is to determine the initial encoding and then transform the characters. This often involves a process called "Unicode conversion," that converts the characters into their corresponding Unicode values.
- Azealia Banks September 9 The Untold Story Of A Fearless Icon
- Unveiling The Mystique Of Harbor Elm Your Ultimate Guide
This situation highlights a common challenge in software development: dealing with character encoding. Different languages and systems employ various methods to store and represent text, which can lead to compatibility problems if not handled correctly. The conversion from one encoding to another is essential for ensuring that text is displayed correctly across various platforms and applications. The need to convert from an encoding like that used in the given example to the more common and universally supported UTF-8 is a necessary operation for a wide range of applications.
The provided examples offer valuable insights into the core issue. The text is represented as a series of Unicode escape sequences, such as \u00f8\u00a7\u00f9\u201e. Unicode is a standard for representing characters from almost all writing systems in the world. The \u escape sequence denotes a Unicode code point, a unique numerical value associated with a specific character. In order to read the text, these unicode escape sequences must be converted into characters. This conversion can be easily achieved using many programming languages.
The provided information mentions a "rest webservice" returning encoded data. The service is returning the Arabic characters which need to be decoded. RESTful web services are designed to communicate via HTTP and often use JSON or XML formats. Ensuring that the correct character encoding is applied at all stages of the data flow is critical. It's necessary that both the web service, and the client application use the same encoding. When an arabic web service returns data, this could include an Arabic string for example. Using the C# code is the solution to decode these types of strings.
- Becker Life And Investment Group Your Gateway To Prosperity And Beyond
- Postech Fashion Inc The Gamechanger In Modern Fashion
The process is crucial. In PDF creation, for example, incorrect character encoding can result in garbled text or missing characters, rendering the document unreadable. It's important that all the elements of the toolchain, from the data source to the PDF generation library, are configured to correctly handle the target encoding.
The examples demonstrate the importance of understanding how character encodings work and how to handle them correctly. The core operation involves a series of steps:
- Identifying the source encoding (the encoding used by the REST service).
- Converting the encoded text into a standard encoding such as UTF-8.
- Using the correct encoding when displaying and processing text in any application.
Using the right tools and a solid understanding of encoding principles can solve most of these problems. Developers face these character encoding challenges in various situations like displaying, processing and storing the information.
Here's a table containing details to help you address the issue with the Arabic text:
Category | Details | Reference |
---|---|---|
Problem | Displaying Arabic text in applications that doesn't support UTF-8 or a different encoding. The text shows up as a series of characters like (\u00f8\u00a7\u00f9\u201e). | Unicode FAQ - UTF-8, UTF-16, UTF-32 & BOM |
Cause | Incorrect character encoding in the source of the data or incorrect decoding. This can happen when the text is stored in a database with an incorrect encoding or when the web service does not specify the correct encoding in the HTTP response. | W3C - Character encodings in HTML |
Solutions - C# |
| Microsoft Docs - Encoding Class |
Example in C# |
| CodeProject - Decoding Unicode Escape Sequences in C# |
Rest Webservice Considerations |
| Microsoft Docs - HttpClient Class |
PDF Generation (iText in Java) |
| iText Documentation |
The process of handling character encodings correctly and the correct approach depend on the tools used and the exact format of the encoded text. The key is to carefully analyze the data, understand the encoding used, and apply the right decoding techniques to transform the encoded data into human-readable and usable Arabic text. The above steps can be used as a basis to troubleshoot and resolve such encoding issues.
The challenges highlighted are common in the world of software development. One should be prepared with the correct set of methods to deal with character encodings in any system. The core problem is the same: ensuring that information is displayed correctly regardless of the system in which it is created or stored.
For example, converting the given sample text to the appropriate character. The code to convert it is simple. It has to recognize the characters, analyze their encoding type and then apply the right technique to convert them to the correct output. The solution would have to involve decoding the Unicode escape sequences. In C#, the System.Text.RegularExpressions.Regex.Unescape method can be used for this. This method converts escape sequences like "\u0041" to the corresponding characters. Then, the developer must choose the correct encoding for the string. For example, to decode it to UTF-8 encoding, developers should use the UTF-8 encoding class.
In the context of creating PDFs with iText, the developer must take into account the character encoding. The first step is to ensure that the font used in the PDF generation supports the Arabic characters. Then the text must be correctly decoded and converted to the correct encoding type, such as UTF-8. This means the source must be correctly interpreted. It must contain the right encoding and is correctly set in the Java code. This is a multistep procedure and will ensure the Arabic text is properly displayed in the PDF.
When it comes to REST web services, a similar set of considerations apply. The REST service returns the data, including the text. The response from the server should include a Content-Type header, specifying the encoding used for the response body. The client application uses the encoding specified in the content type or it has to decode the data. If the data received is in a form that is not supported by the application, the application must convert it to a supported format.
Another factor is that the data may come from a database or flat text file. The same principles apply here, the file must be opened using the proper encoding, or the encoding has to be recognized. In many cases, it is necessary to configure the software correctly to handle the specific encoding. For example, in Java, you would specify the encoding when reading a file to ensure that characters are interpreted correctly.
Another example is a website with a problem with the encoding: The website displays characters as \u00f8\u00b3\u00f9\u201e\u00f8\u00a7\u00f9\u0161\u00f8\u00af\u00f8\u00b1. The solution involves ensuring that the correct character encoding is set in the HTML of the web pages, the servers configuration, and the database where the data is stored. This means setting the tag in the HTML and configuring the server to send the correct Content-Type headers. The same thing applies to the database settings as well, the developer should make sure that the data is stored using UTF-8.
The Unicode standard provides a comprehensive way to represent text in any language. However, implementing that standard correctly in software is still a complex matter. Different software components must use the correct encodings throughout the process. Understanding and applying these concepts is crucial for dealing with internationalized content in modern software applications.
The Unicode standard and its encodings are vital for digital communication. It enables the display of characters from all languages and writing systems worldwide. The challenge in handling text from different languages arises when working with data from various sources. For instance, a service might return text in a specific encoding, such as UTF-8, but the receiving application or system may not interpret it correctly.
Consider a scenario where a web service delivers Arabic text, but the display in the application is garbled. This issue indicates a mismatch in encoding. The solution involves identifying the original encoding of the data, such as UTF-8, and converting the encoded text into a format compatible with the application. This might require using appropriate encoding classes or conversion methods in programming languages like C#.
For developers creating PDFs, the correct handling of character encoding is crucial. It ensures that the text appears correctly in the document. This entails selecting fonts that support the required characters, correctly setting the encoding parameters within the PDF generation library and ensuring the source text is appropriately decoded before insertion into the PDF. Failing to do so can result in unreadable content or missing characters.
Dealing with character encodings involves steps for effective management. The first step involves identifying the source encoding of the data. Determine the original character encoding used by the service, database, or file. Once determined, conversion to UTF-8 is often the next step. The conversion ensures universal support and eliminates encoding-related display issues across different systems. In C#, tools like System.Text.Encoding and System.Text.RegularExpressions.Regex.Unescape are useful for these operations.
Web services also need specific considerations. The web service has to provide the correct Content-Type header in its HTTP response, indicating the encoding of the response body (e.g., application/json; charset=utf-8). The client application then needs to recognize and handle this header correctly, using it to decode the data. If a mismatch occurs, the client application might display the text incorrectly.
For those working with databases, the correct configuration of the database and tables is vital. This includes setting the character set and collation settings. The same principles apply to reading text files: using the correct encoding when opening and reading the file.
In essence, character encoding is fundamental. It impacts the accuracy and reliability of text-based systems. Understanding the concepts, adopting the best practices, and using the appropriate tools can help developers avoid and fix character encoding issues. When applied correctly, they ensure data is handled and displayed in the correct way.
The examples provided, from dealing with REST web services returning encoded data to creating PDFs and working with databases, share a common thread. They all demonstrate the need to correctly handle character encodings. It's about ensuring that the original meaning of the text is preserved throughout the process. It requires a meticulous approach, a solid understanding of encodings, and using the right tools for the job.
In summary, managing character encodings is essential for anyone working with digital text. The key is to correctly identify the source encoding. It's then essential to ensure the correct decoding methods are employed, which varies depending on the programming language and libraries used. Properly handling character encodings guarantees that textual content is accurately displayed and processed. It also prevents data corruption and ensures that communications remain clear.
- Lauryn Hill Locs The Ultimate Guide To Her Iconic Style
- Coreano Flequillo Asiatico The Ultimate Guide To This Trendy Hairstyle
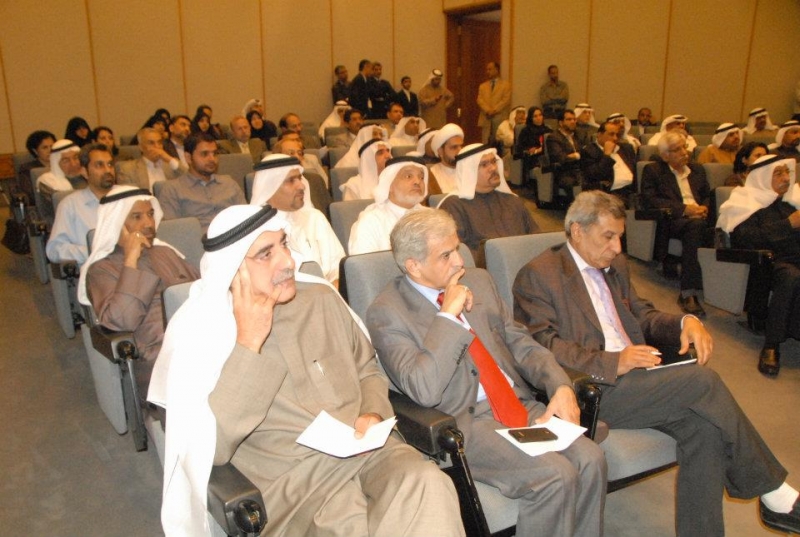
